A Practical Guide to Scraping Airtable Data with Chrome DevTools and JavaScript
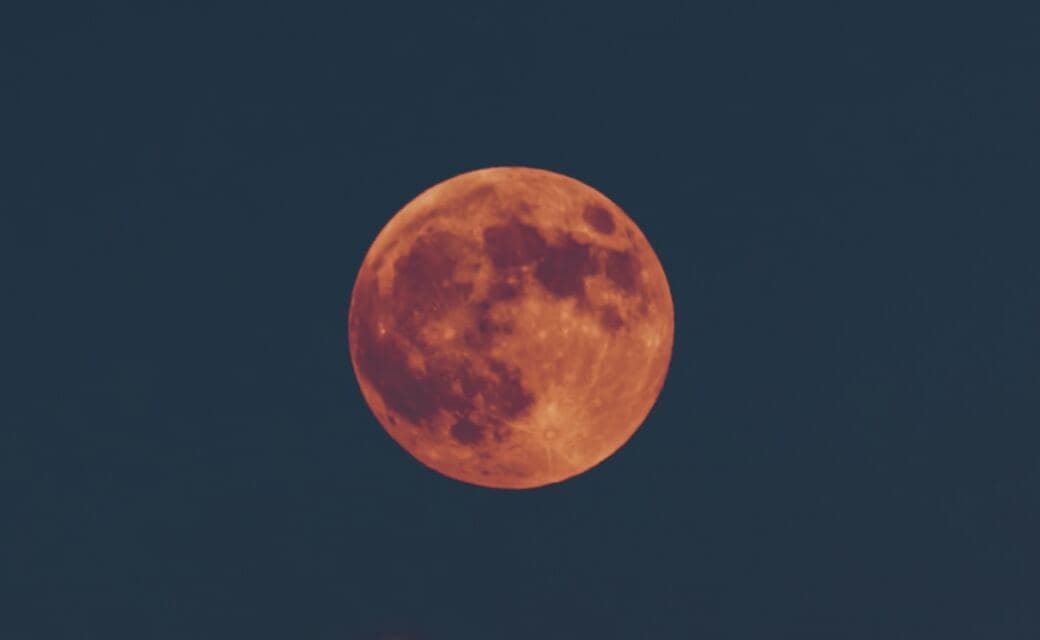
In this guide, we will explore a practical and efficient method to extract data from Airtable using Chrome DevTools and a bit of JavaScript. This approach allows us to access the data directly from the browser and convert it into a CSV format, ready for use in other applications.
Extracting Data Using Chrome DevTools
To extract data from Airtable, we will follow these steps:
- Open the Airtable in Chrome and access Chrome DevTools (Ctrl+Shift+I / Cmd+Shift+I)
- Navigate to the Network tab and refresh the page
- Identify the request containing the data, right-click the response, and store it as a global variable (e.g.,
temp1
)
JavaScript Code for Data Conversion
With our data stored in a global variable, we can now use the following JavaScript code to access and convert it into a more usable format:
let columns = temp1.table.columns;
function getData(row) {
return columns.reduce((acc, column) => {
if (row.cellValuesByColumnId[column.id]) {
acc[[column.name]] = row.cellValuesByColumnId[column.id];
}
return acc;
}, {});
}
let data = temp1.table.rows.map((row) => {
return getData(row);
});
function convertToCSV(data) {
const header = Object.keys(data[0]).join(',') + '\n';
const rows = data
.map((row) => {
return Object.values(row)
.map((value) => `"${value}"`)
.join(',');
})
.join('\n');
return header + rows;
}
const csvData = convertToCSV(data);
copy(csvData); // Copies the entire CSV data to clipboard
Executing the Code
To execute our JavaScript code and extract the data:
- Run the JavaScript code in the Console tab of Chrome DevTools
- The entire CSV data will be copied to your clipboard, ready for use in other applications
Conclusion
This practical approach to scraping Airtable data using Chrome DevTools and JavaScript provides an efficient method for extracting data directly from the browser. By converting the data into a CSV format, we can easily use it in other applications or for further analysis.